<?php
/*
insert.php
return x,y : x=idkey=first idtable insert, y=last idtable inserted
*/
require_once('call_class.php');
$call = new call_class();
// insert_1 : first insert with common idkey automatically calculated
$call->cmd = "
<record>
<stmt>insert</stmt>
<field>col_user,col_key,col_value</field>
<paramf>User_1</paramf>
<paramf>name</paramf>
<paramf>Tom</paramf>
</record>
<record>
<field>col_key, col_value</field>
<paramf>birthday</paramf>
<paramf>1966-04-30</paramf>
</record>
<record>
<field>col_key,col_value</field>
<paramf>city</paramf>
<paramf>San Francisco</paramf>
</record>
";
$Res = $call->call_curl();
if ( substr($Res, 0, 4) != "#ERR" ) {
$IdKey = explode(",", $Res)[0];
$IdTable = explode(",", $Res)[1];
echo "- Insert of 3 records from ".$IdKey." to ".$IdTable.", idkey=".$IdKey."<br />";
}else{
echo "error in insert_1...".$Res;
die();
}
// insert_2 : insert with idkey searched with select (insert at a later time with association to a group of records)
//
// search idkey for next associated inserts
$call->cmd = "
<record>
<stmt>select</stmt>
<field>col_idkey</field>
<Where>col_user=? and col_key=? and col_value=?</Where>
<paramW>User_1</paramW>
<paramW>name</paramW>
<paramW>Tom</paramW>
<OtherCmd>LIMIT 1</OtherCmd>
<ReturnSelect>STRING</ReturnSelect>
<RowHeading>N</RowHeading>
</record>
";
$Res = $call->call_curl();
if ( substr($Res, 0, 4) != "#ERR" ) {
$IdKey = $Res; // return only single data (col_idkey)
$call->cmd = "
<record>
<stmt>insert</stmt>
<field>col_user,col_idkey,col_key,col_value,col_file</field>
<paramf>User_1</paramf>
<paramf>".$IdKey."</paramf>
<paramf>text_file</paramf>
<paramf>sample.txt</paramf>
<paramf>text in sample file...</paramf>
</record>
<record>
<field>col_user,col_idkey,col_key,col_value,col_file</field>
<paramf>User_1</paramf>
<paramf>".$IdKey."</paramf>
<paramf>photo</paramf>
<paramf>photo_tom.jpg</paramf>
<paramf>".file_get_contents("photo_tom.jpg")."</paramf>
</record>
";
$Res = $call->call_curl();
if ( substr($Res, 0, 4) != "#ERR" ) {
$IdKey = explode(",", $Res)[0];
$IdTable = explode(",", $Res)[1];
echo "- Insert of 2 associated with idkey=".$IdKey."<br />";
}else{
echo "error in insert_2...".$Res;
die;
}
}else{
echo "error in Select...".$Res;
die;
}
//EOF
?>
Nella prima chiamata ci sono 3 blocchi <record> che eseguiranno 3 insert relazionati alla stessa idkey generata automaticamente e che sarà assegnata uguale alla col_idtable
Ritorna questo risultato :


Poi si esegue una ‘select…’ per ricavare la idkey da relazionare ai successivi 2 ‘insert’. Il primo ‘insert’ memorizza un file di testo con il contenuto esplicito, la seconda ‘insert’ memorizza il contenuto di un file immagine.
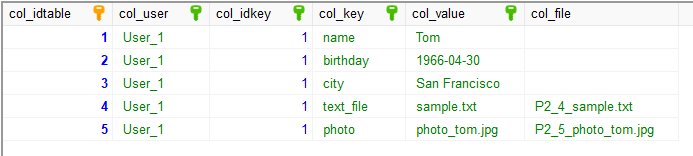